1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | public class FindOvelsConsonents { public static void main(String[] args) { // TODO Auto-generated method stub FindOvelsConsonents o = new FindOvelsConsonents(); o.findvowelsAndConsonents("NIHAL Is my Name"); o.findvowelsAndConsonentsUsingContains("NIHAL IS MY NAME"); } int count = 0, count1; // ===================Method: 1 ====================== void findvowelsAndConsonents(String str) { String s = str.toLowerCase(); System.out.println(s); for (int i = 0; i < s.length(); i++) { if (s.charAt(i) == 'a' || s.charAt(i) == 'e' || s.charAt(i) == 'i' || s.charAt(i) == 'o' || s.charAt(i) == 'u') { count++; } else if (s.charAt(i) == ' ') { } else { count1++; } } System.out.println("Ovels are: " + count + ", and Consonents are: " + count1); } // ========================Method:2 USING CONTAINS================== int vowels; int conso; void findvowelsAndConsonentsUsingContains(String str) { // int[] i = new int[256]; String strV = "aeiouAEIOU"; for (int j = 0; j < str.length(); j++) { if (strV.contains(Character.toString(str.charAt(j)))) { // Main Logic vowels++; // Incrementng vowels count } else if (str.charAt(j) == ' ') { } else { conso++; // Incrementng Consonents count } } System.out.println("Ovels are: " + vowels + " Consonents are: " + conso); } } |
Find Vowels and Consonants in given String
Collections Interview Questions(Theoretical)
1. What is HashMap and Map?
Map is an interface. Contains methods to manipulate Key-Value based collections. The main methods of Map interface are put(K,V), get(K), Collection<V> values(), Set<K> keySet(), containsKey(), containsValue()
HashMap is one of implementations of the Map interface based on hashcodes of objects used as keys.
2. Difference between HashMap and HashTable? Can we make hashmap synchronized?
Both implement Map interface. HashTable is synchronized. It is recommended to use HashMap wherever possible. HashTable doesn't allow null keys and values. HashMap allows one null key and any number of null values.
We can make it synchronized
Map m = Collections.synchronizedMap(new HashMap());
3. Difference between Vector and ArrayList?
Both implement List interface. ArrayList is not synchronized.
4. List vs Set vs Map. Purposes and definitions.
All three are interfaces.
List -- storing values in specified order. Provides methods to get the element by its position get(i), finding the element, ListIterator. Known implementations: ArrayList, Vector, LinkedList. The list should be used when the order in which the elements are stored matters.
Set -- storing only different objects and at most one null element. Known implementations: TreeSet (iterate over the elements in order defined by Comparator, or if the elements implement comparable; provides log(n) performance for basic operations), HashSet -- stores values in buckets defined by their hashcodes. Each bucket is a singly linked list. Provides constant time performance for basic operations. LinkedHashSet
Map -- for storing key-value pairs. The map cannot contain duplicate keys. Provides three collection views: a set of keys, a collection of values, set of key-value mappings. Know implementations HashMap, EnumMap, TreeMap, LinkedHashMap, WeakHashMap.
5. What is an Iterator?
It is an interface that contains three methods: next(), boolean hasNext(), void remove()
It allows iterating over the collection
If the class implements iterator then it can be used in foreach loop
6. Pros and cons of ArrayList and LinkedList
ArrayList -- fast random access.
LinkedList -- slow random access. Implements Queue interface. Fast deletion of the element.
If lots of random reads are anticipated use ArrayList.
If lots of iterations over the whole list and lots of add/delete -- use LinkedList.
7. TreeSet vs LinkedHashSet
LinkedHashSet is backed by LinkedHashMap. LinkedHashMap is backed by doubly linked list to enforce ordering on the elements contained in the Map.
If the ordering of the elements in the Set matters to you but you don't want to use a comparator you may use LinkedHashSet since it will enforce order in which the elements were added to the set. Otherwise, use TreeSet
8. Differences between Hashtable, ConcurrentHashMap and Collections.synchronizedMap()
ConcurrentHashMap allows concurrent modification of the Map from several threads without the need to block them. Collections.synchronizedMap(map) creates a blocking Map which will degrade performance, albeit ensure consistency (if used properly).
Use the second option if you need to ensure data consistency, and each thread needs to have an up-to-date view of the map. Use the first if performance is critical, and each thread only inserts data to the map, with reads happening less frequently.
9. How are hash codes computed?
if hashCode() method is defined then it is called to calculate the hashcode
if it's not defined the default implementation in Object class does the following:
public int hashCode() {
return VMMemoryManager.getIdentityHashCode(this);
}
What is the significance of ListIterator? What is the difference b/w Iterator and ListIterator?
ListIterator allows to perform iteration both ways (first-->last and last-->first)
From JavaDoc: ListIterator is an iterator for lists that allows the programmer to traverse the list in either direction, modify the list during iteration, and obtain the iterator's current position in the list
10. Is it possible that hash code is not unique?
It's totally possible. Actually, a totally valid hashCode() function could look like this
int hashCode(){ return 57;
What are the advantages of ArrayList over arrays?
1. ArrayList comes with a number of utility methods (e.g. contains, remove, addAll)
2. Type safety
3. Dynamic sizing
On the other hand, arrays are a little bit faster and take less memory (packing). Arrays are also able to contain values of primitive types while ArrayList can only contain Objects.
11. The principle of storing data in a hashtable
HashSet. add(element) -> element.hashCode() -> mod bucketsCount -> store
HashMap. add(key, value) -> key.hashCode() -> mod bucketsCount -> store(value)
12. Can we put two elements with equal hash code to one hashmap?
Yes.The hash code of the objects doesn't matter, Only the hashcode of keys. But even if you want to put the keys with the same hashcode, it will be ok, since it just means that key-value pairs will be put into the same bucket
13. Iterator and modification of a List. ConcurentModificationException.
The iterators returned by this class's iterator method are fail-fast: if the set is modified at any time after the iterator is created, in any way except through the iterator's own remove method, the iterator will throw a ConcurrentModificationException. So, in the face of concurrent modification, the iterator fails quickly and cleanly, rather than risking arbitrary, non-deterministic behavior at an undetermined time in the future.
Note that the fail-fast behavior of an iterator cannot be guaranteed as it is, generally speaking, impossible to make any hard guarantees in the presence of unsynchronized concurrent modification. Fail-fast iterators throw ConcurrentModificationException on a best-effort basis. Therefore, it would be wrong to write a program that depended on this exception for its correctness: the fail-fast behavior of iterators should be used only to detect bugs.
Merge Two Sorted Arrays without extra space
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 | //Merge 2 sorted Arrays where Bigger arrays have a space for smaller Array //Example: arr1[] = {2,3,4,0,0,0,} //arr2[] = {5,6,7} Array-2 size is 3, which can be places at array-1 import java.util.Arrays; public class Merge2SortedArrays { public static void main(String[] args) { Merge2SortedArrays o = new Merge2SortedArrays(); int[] arr1 = { 1, 2, 2, 13, 0, 0, 0, 0 }; int[] arr2 = { 1, 3, 8, 9 }; o.getSortedArray(arr1, arr2); } /* * Taking array1(element) and array1(element) comparing if arr1 element is * lesser to arr2 element increment if arr1 element is greater to arr2 element * swap and sort array2 * * Now iterate throught the total array and place sorted 2nd array into 1st * array an print * */ public void getSortedArray(int[] arr1, int[] arr2) { int arr1Begin = 0; int jarr2Begin = 0; int temp = 0; int findLength = (arr1.length) - arr2.length; System.out.println(findLength); for (int k = 0; k < findLength; k++) { if (arr1[arr1Begin] < arr2[jarr2Begin]) { arr1Begin++; } if (arr1[arr1Begin] >= arr2[jarr2Begin]) { temp = arr1[arr1Begin]; arr1[arr1Begin] = arr2[jarr2Begin]; arr2[jarr2Begin] = temp; arr1Begin++; Arrays.sort(arr2); } } System.out.println("1st sorted array: " + Arrays.toString(arr1)); System.out.println("2nd array is: " + Arrays.toString(arr2)); System.out.println("*********Merging 2 sorted Arrays**************"); int temp1 = 0; int p = 0; for (int m = findLength; m < arr1.length; m++) { temp1 = arr1[findLength]; arr1[findLength] = arr2[p]; arr2[p] = temp1; p++; findLength++; } System.out.println("Final Sorted Array: " + Arrays.toString(arr1)); } } |
REST Introduction
Rest: (Representational State Transfer)
- It is an architectural style for developing web-services.
- It is popular (because it is simple). It supports multiple formats like JSON, Text, XML..etc.
- Rest is also language-independent architectural style, most of the REST-based application can be written in any language (Java, Angular JS, python) as long as the language support web-based request using HTTP.
- Reason: The reason why REST is popular is supporting multiple programming languages and multiple formats. Before REST developers used SOAP which mainly supports XML and had restrictions. Whereas, REST offers much better style for an approach.
REST: (Main operations)
PUT: Used to create a new resource.
POST: Used to update an existing resource or create a new resource.
GET: Provides a read only access to a resource.
DELETE: Used to remove a resource.
Print ZigZag String
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | public class ZigZazString { public static void main(String[] args) { // TODO Auto-generated method stub ZigZazString o = new ZigZazString(); o.doZig("NihalKurre"); // Passing string to the method } void doZig(String str) { for (int i = 0; i < str.length(); i++) { // Iterating through the string length if ((i % 4) == 0) { System.out.print(str.charAt(i) + " "); } } System.out.println(); for (int i = 0; i < str.length(); i++) { if ((i % 2) != 0) { System.out.print(str.charAt(i) + " "); } } System.out.println(); String s = str.substring(2, str.length()); // Taking the 2 to rest of the element in given string for (int i = 0; i < s.length(); i++) { if ((i % 4) == 0) { System.out.print(s.charAt(i) + " "); } } } } |
Find Highest Number In an Array
public class FindHghestNumberInArray {
public static void main(String[] args) {
// TODO Auto-generated method stub
FindHghestNumberInArray f= new FindHghestNumberInArray();
int[] arr ={1,2,3,4,5,4,2};
f.findHighest(arr);
}
public void findHighest(int[] arr) {
for(int i=1; i< arr.length; i++) {
if(arr[i] < arr[i-1]) {
System.out.print(arr[i-1]+ " ");
break;
}
}
}
}
Collections
Collection Framework:
what are Collections.?
-It is a framework which provides the architecture to store and manipulate the group of objects.
- The collection is a container in which group of elements are combined together to form a single unit.
Collection in Java classified into 3 categories:
- Interface: It is an abstract data-type(ADT) which represents the collection.
- Implementation: It is a concrete implementation of the interface.
- Algorithms: It contains methods which perform useful computations like searching and sorting.
The following ways collections are classified:
- Set: It is special kind of interface which does not allow duplicates.
- List: It allows duplicates and maintains the order
- Map: It is key, value pair.
Collection in Java classified into 3 categories:
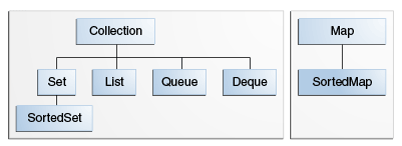
SET in Detail : (java.util )
- It is special kind of interface which does not allow duplicates.
- Set is an interface which extends Collection.
- It is an unordered collection of objects in which duplicate values cannot be stored.
Syntax: Set <Object> someName= new HashSet<Object>();
Example: Set <String> s= new HashSet<String>();
- It is special kind of interface which does not allow duplicates.
- Set is an interface which extends Collection.
- It is an unordered collection of objects in which duplicate values cannot be stored.
Syntax: Set <Object> someName= new HashSet<Object>();
Example: Set <String> s= new HashSet<String>();
METHODS:
boolean add(Object o)--->It is used to add the specified element to this set if it is not already present.
Eg: s.add("Nihal");
s.add("Kurre");
boolean remove(Object o) ---> It is used to remove the specified element from this set if it is present.
Eg: s.remove("Nihal");
void clear()---> It is used to remove all of the elements from this set.
Eg: s.clear();
int size()---> It is used to return the number of elements in this set.
Eg: s.size();
boolean contains(Object o)---> It is used to return true if this set contains the specified element.
Eg: s.contains("Nihal");
boolean isEmpty()---> It is used to return true if this set contains no elements.
Eg: s.isEmpty();
Object clone()---> It is used to return a shallow copy of this HashSet instance: the elements themselves are not cloned.
Iterator iterator()---> It is used to return an iterator over the elements in this set.
HashSet: Does not sort.can contain null values.
LinkedHashSet: Takes the insertion order. It can have Null values
TreeSet: It is sorted and null value depends
ConcurrentSkip List set: It is sorted and weakly consistent. It does not have null values.
int size()---> It is used to return the number of elements in this set.
boolean contains(Object o)---> It is used to return true if this set contains the specified element.
Object clone()---> It is used to return a shallow copy of this HashSet instance: the elements themselves are not cloned.
Iterator iterator()---> It is used to return an iterator over the elements in this set.
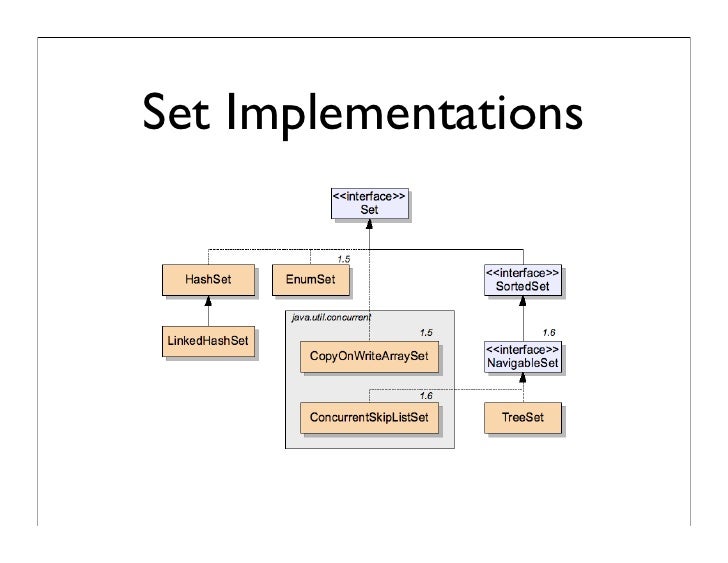
List In Detail :
- It is special kind of interface which does not allow duplicates.
- Set is an interface which extends Collection.
- It is an unordered collection of objects in which duplicate values cannot be stored.
Syntax: List<Object> someName= new HashTable<Object>();
Example: List<String> l= new HashTable<String>();
- It is special kind of interface which does not allow duplicates.
- Set is an interface which extends Collection.
- It is an unordered collection of objects in which duplicate values cannot be stored.
Syntax: List<Object> someName= new HashTable<Object>();
Example: List<String> l= new HashTable<String>();
METHODS:
void add(int index,Object element)--->It is used to insert an element into the invoking list at the index passed in the index.
Eg: l.add("Nihal");
boolean addAll(int index,Collection c)--->It is used to insert all elements of c into the invoking list at the index passed in the index.
object get(int index)--->It is used to return the object stored at the specified index within the invoking collection.
object set(int index,Object element)---> It is used to assign an element to the location specified by index within the invoking list.
object remove(int index)---> It is used to remove the element at position index from the invoking list and return the deleted element.
ListIterator listIterator()---> It is used to return an iterator to the start of the invoking list.
ListIterator listIterator(int index)---> It is used to return an iterator to the invoking list that begins at the specified index
void add(int index,Object element)--->It is used to insert an element into the invoking list at the index passed in the index.
Eg: l.add("Nihal");
boolean addAll(int index,Collection c)--->It is used to insert all elements of c into the invoking list at the index passed in the index.
object get(int index)--->It is used to return the object stored at the specified index within the invoking collection.
object set(int index,Object element)---> It is used to assign an element to the location specified by index within the invoking list.
object remove(int index)---> It is used to remove the element at position index from the invoking list and return the deleted element.
ListIterator listIterator()---> It is used to return an iterator to the start of the invoking list.
ListIterator listIterator(int index)---> It is used to return an iterator to the invoking list that begins at the specified index
Map In Detail:
- The java.util.Map interface represents a mapping between a key and a value.
- A Map cannot contain duplicate keys and each key can map to at most one value.
- Some implementations allow null key and the null value (HashMap and LinkedHashMap) but some do not (TreeMap).
Why Map:
A map of zip codes and cities.
A map of managers and employees. Each manager (key) is associated with a list of deatils of employees (value) he manages.
A map of classes and students. Each class (key) is associated with a list of students values.
Best: Map is best for searching.
It implements a serializable and clonable interface.
Syntax: Map<Object, Object>someName= new HashMap<Object, Object>();
Eg: Map<Integer, Integer>map= new HashMap<Integer, Integer>();
- The java.util.Map interface represents a mapping between a key and a value.
- A Map cannot contain duplicate keys and each key can map to at most one value.
- Some implementations allow null key and the null value (HashMap and LinkedHashMap) but some do not (TreeMap).
Why Map:
A map of zip codes and cities.
A map of managers and employees. Each manager (key) is associated with a list of deatils of employees (value) he manages.
A map of classes and students. Each class (key) is associated with a list of students values.
METHODS:
Object put(Object key, Object value)--->It is used to insert an entry in this map.
void putAll(Map map)---> It is used to insert the specified map in this map.
Object remove(Object key)---> It is used to delete an entry for the specified key.
Object get(Object key)---> It is used to return the value for the specified key.
boolean containsKey(Object key)---> It is used to search the specified key from this map.
Set keySet()---> It is used to return the Set view containing all the keys.
Set entrySet()---> It is used to return the Set view containing all the keys and values.
Object put(Object key, Object value)--->It is used to insert an entry in this map.
void putAll(Map map)---> It is used to insert the specified map in this map.
Object remove(Object key)---> It is used to delete an entry for the specified key.
Object get(Object key)---> It is used to return the value for the specified key.
boolean containsKey(Object key)---> It is used to search the specified key from this map.
Set keySet()---> It is used to return the Set view containing all the keys.
Set entrySet()---> It is used to return the Set view containing all the keys and values.
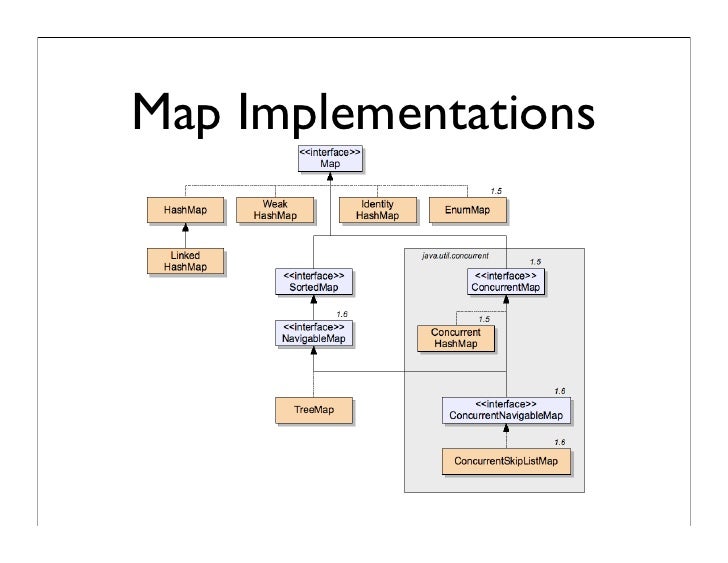
Inserting Elements In Sorted Array (2methods)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 | package DS; //https://leetcode.com/problems/search-insert-position/description/ //Given a sorted array and a target value, return the index if the target is found. //If not, return the index where it would be if it were inserted in order. public class InsertingElementInSortedArray { public static void main(String[] args) { // TODO Auto-generated method stub InsertingElementInSortedArray o = new InsertingElementInSortedArray(); int[] arr = { 1, 2, 5, 6, 10, 12, 14, 15, 16 }; o.doMethod(arr, 3); } void doMethod(int[] arr, int key) { int low = 0; int high = arr.length; int mid = (low + high) / 2; if (arr.length == 0) { return; } if (key < arr[mid]) { sequentialSearch(low, mid, arr, key); } if (key > arr[mid]) { sequentialSearch((mid), high, arr, key); } } void sequentialSearch(int low, int high, int[] arr, int key) { for (int i = low + 1; i < high; i++) { if (key == arr[i - 1]) { System.out.println("found at: " + i); break; } if (key == arr[i]) { System.out.println(" Found at: " + i); break; } if ((key < arr[i]) && (key > arr[i - 1])) { System.out.println(" Should be between: " + arr[i - 1] + " " + arr[i]); } } } } // Output: Should be between: 2 5 ================================= public class InsertingElementInSortedArrTREEMAP { public static void main(String[] args) { // TODO Auto-generated method stub InsertingElementInSortedArrTREEMAP o = new InsertingElementInSortedArrTREEMAP(); int[] arr = { 2, 4, 5, 6, 7, 10, 20, 30 }; o.usingTreeMap(arr, 22); } void usingTreeMap(int[] arr, int cc) { Map map = new TreeMap(); Integer count = 0; // int stream or Array.stream= java.util.Arrays.stream(int[]) // boxed= java.util.stream.IntStream.boxed() // : := Integer[] java.util.function.IntFunction.apply(int value) // STREAMS: https://www.geeksforgeeks.org/streams-arrays-java-8/ // https://www.youtube.com/watch?v=gFu3TJhog7E Integer[] what = Arrays.stream(arr).boxed().toArray(Integer[]::new); // important for (int i : what) { map.put(i, count); count++; } System.out.println(map.toString()); System.out.println("========================"); if (map.containsKey(cc)) { System.out.println(map.get(cc)); } else { map.put(cc, count); System.out.println("after adding: " + map); System.out.println(" ============================"); if (map.containsKey(cc)) { System.out.println("Element shoud be present between Vales: " + (map.get(cc) - 1) + " index and " + (map.get(cc) + 1)); } } } } // Method: 2 Logic by Santosh // Output: // {2=0, 4=1, 5=2, 6=3, 7=4, 10=5, 20=6, 30=7} ======================== // after adding: {2=0, 4=1, 5=2, 6=3, 7=4, 10=5, 20=6, 22=8, 30=7} ============================ // Element shoud be present between Vales: 7 index and 9 |
Find Pair in array equal to target
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | import java.util.HashSet; import java.util.Set; class FindPairs { public static void main(String[] args) { FindPairs obj = new FindPairs(); int[] arr1 = { 22, 1, 2, 3, 4, 5, 6, -1, 7, 9, 3, 15 }; obj.getPairs1(arr1, 6); } private void getPairs1(final int[] input, int sum) { System.out.println(" Array is: 22, 1, 2, 3, 4, 5, 6, -1, 7, 9, 3, 15 and sum=6"); Set<integer> set = new HashSet(input.length); for (int i = 0; i < input.length; i++) { if (set.contains(sum - input[i])) { System.out.println("(" + input[i] + ", " + (sum - input[i]) + ") "); } else { set.add(input[i]); } } } } |
Subscribe to:
Posts (Atom)